반응형
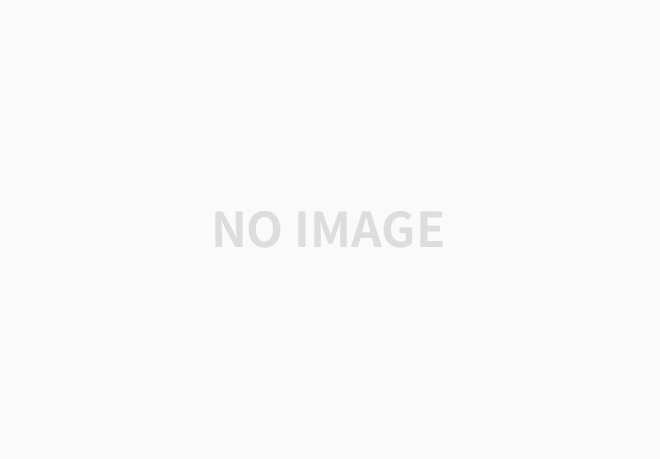
FixedVector.h
#pragma once
namespace samples
{
template<typename T, size_t N>
class FixedVector
{
public:
FixedVector();
bool Add(const T& data);
size_t GetSize() const;
size_t GetCapacity() const;
private:
size_t mSize;
T mArray[N];
};
template<typename T, size_t N>
FixedVector<T, N>::FixedVector()
: mSize(0)
{
}
template<typename T, size_t N>
size_t FixedVector<T, N>::GetSize() const
{
return mSize;
}
template<typename T, size_t N>
size_t FixedVector<T, N>::GetCapacity() const
{
return N;
}
template<typename T, size_t N>
bool FixedVector<T, N>::Add(const T& data)
{
if (mSize >= N)
{
return false;
}
mArray[mSize++] = data;
return true;
}
}
FixedVectorExample.cpp
#include <iostream>
#include "FixedVector.h"
#include "FixedVectorExample.h"
#include "IntVector.h"
using namespace std;
namespace samples
{
void FixedVectorExample()
{
FixedVector<int, 3> scores;
scores.Add(10);
scores.Add(50);
cout << "scores - <Size, Capacity>: " << "<" << scores.GetSize()
<< ", " << scores.GetCapacity() << ">" << endl;
FixedVector<IntVector, 5> intVectors;
intVectors.Add(IntVector(2, 5));
intVectors.Add(IntVector(4, 30));
intVectors.Add(IntVector(22, 3));
cout << "intVectors - <Size, Capacity>: " << "<" << intVectors.GetSize()
<< ", " << intVectors.GetCapacity() << ">" << endl;
FixedVector<IntVector*, 4> intVectors2;
IntVector* intVector = new IntVector(3, 2);
intVectors2.Add(intVector);
cout << "intVectors2 - <Size, Capacity>: " << "<" << intVectors2.GetSize()
<< ", " << intVectors2.GetCapacity() << ">" << endl;
delete intVector;
}
}
고정형 사이즈 벡터를 만드는 방법을 템플릿을 활용하여 구현한 예제입니다. 이처럼 STL 고정 라이브러리에 필요한 기능들을 개발자가 추가하여서 활용할 수 있다는 것을 알 수 있었습니다.
원본 코드는 아래 경로에서 확인할 수 있습니다.
https://github.com/POCU/COMP3200CodeSamples/blob/master/09/FixedVector.h
POCU/COMP3200CodeSamples
Code samples for COMP3200. Contribute to POCU/COMP3200CodeSamples development by creating an account on GitHub.
github.com
반응형
'SW > C++' 카테고리의 다른 글
C++ : 템플릿 : 특수화, 장점과 단점, 베스트 프랙티스 (0) | 2020.04.24 |
---|---|
C++ : 템플릿 함수 : Math 예제 구현해보기 (0) | 2020.04.23 |
C++ : 클래스 템플릿 : 예제, 사용법, 구현 방법, 추천 (0) | 2020.04.21 |
C++ : 템플릿 프로그래밍 : 기본 개념, 예제, 활용방법, 주의사항 (0) | 2020.04.20 |
C++ : 가변 인자 템플릿 : 장점, 사용법, 예제, 개념 (0) | 2020.04.19 |