반응형
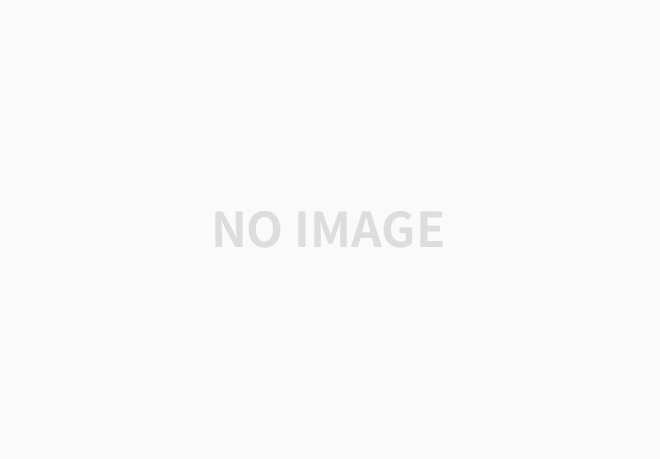
std::unique_ptr
포인터 대신 아주 많이 활용되고 있습니다. 직접 메모리 관리하는 것 만큼 빠릅니다. Resource Acquisition is initialization 원칙에 잘 들어맞습니다. 자원 할당은 개체의 수명과 관련되어 있습니다. 생성자에서 new를 하고 소멸자에서 delete를 해주어야하는데 유니크 포인터 멤버 변수가 알아서 동작하여 줍니다. 따라서, 실수하기 어려우며, 다양한 곳에서 활용하여도 좋습니다.
예제
#include <memory>
#include <iostream>
#include "UsingUniquePointersExample.h"
#include "MyVector.h"
using namespace std;
namespace samples
{
void UsingUniquePointersExample()
{
unique_ptr<int> num1 = make_unique<int>(10);
unique_ptr<char> char1 = make_unique<char>('d');
cout << *num1 << endl;
cout << *char1 << endl;
unique_ptr<MyVector> myVector = make_unique<MyVector>(3, 5);
cout << "X: " << myVector->GetX() << ", Y: " << myVector->GetY() << endl;
// Compiler error
// unique_ptr<MyVector> copyOfMyVector1 = myVector;
// unique_ptr<MyVector> copyOfMyVector2(myVector);
myVector.reset(new MyVector(1, 5));
cout << "X: " << myVector->GetX() << ", Y: " << myVector->GetY() << endl;
num1.reset();
myVector = nullptr;
unique_ptr<char> char2(move(char1));
// Run-time error
//cout << "Char1: " << *char1 << endl;
cout << "Char2: " << *char2 << endl;
const unique_ptr<float> float1 = make_unique<float>(2.0f);
// Compile error
// unique_ptr<float> float2(move(float1));
//float1.reset(new float(1.0f));
}
}
원본 코드는 아래 경로에서 확인할 수 있습니다. 유니크 포인터는 기존 C++의 치명적인 단점을 완화시켜주는 녀석이라는 것을 알게 되었습니다. 포인터를 사용하는 곳에서는 가능한 많이 사용하여도 무방할 것 같습니다.
https://github.com/POCU/COMP3200CodeSamples/blob/master/11/UsingUniquePointersExample.cpp
POCU/COMP3200CodeSamples
Code samples for COMP3200. Contribute to POCU/COMP3200CodeSamples development by creating an account on GitHub.
github.com
반응형
'SW > C++' 카테고리의 다른 글
C++ : shared_ptr : 개념, 예제, 사용법, 구현 (0) | 2020.05.08 |
---|---|
C++ : 자동 메모리 관리, 가비지 컬렉션, 참조 카운트 : 개념, 관계, 장단점 (0) | 2020.05.07 |
C++ : unique_ptr : reset, get, release, move, 복사 : 개념, 예제 (0) | 2020.05.05 |
C++ : unique_ptr : 유니크 포인터 개념, 필요성, 장점, 활용법 (0) | 2020.05.04 |
C++ : std::array : 개념, 장점, 단점, 필요성, 활용성, 예제, 구현 (0) | 2020.05.03 |