반응형
%matplotlib inline
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from keras.layers import *
from keras.models import *
from keras.utils import *
from sklearn.preprocessing import *
import seaborn as sns
먼저 필요한 라이브러리들을 임포트 합니다.
from keras.datasets import cifar10
(X_train, Y_train) , (X_test, Y_test) = cifar10.load_data()
X_train.shape
fig = plt.figure(figsize=(20,5))
for i in range(36):
ax = fig.add_subplot(3, 12, i+1, xticks=[], yticks=[])
ax.imshow(X_train[i])
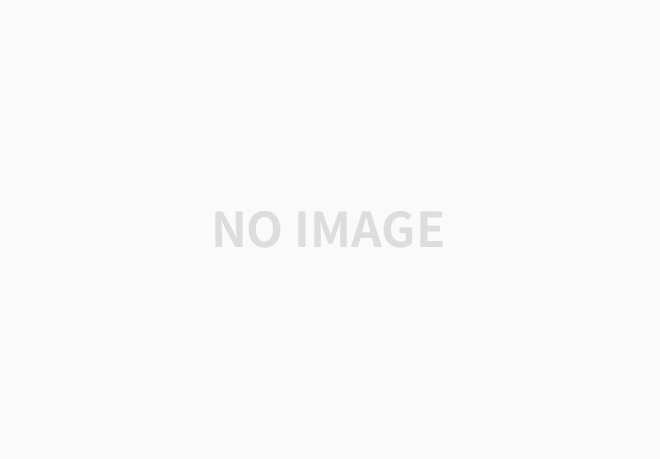
사용할 데이터를 로드하고, 어떠한 데이터인지 확인합니다. 위와 같은 데이터들이라는 것을 확인할 수 있습니다.
X_train = X_train/255.0
X_test = X_test /255.0
데이터를 정규화합니다.
Y_train = to_categorical(Y_train)
Y_test = to_categorical(Y_test)
출력 데이터는 다음과 같이 전처리합니다.
model = Sequential()
model.add(Conv2D(filters=16, kernel_size=4, padding='same', strides=1, activation='relu', input_shape=(32,32,3)))
model.add(MaxPool2D(pool_size=2))
model.add(Conv2D(filters=32, kernel_size=4, padding='same', strides=1, activation='relu'))
model.add(MaxPool2D(pool_size=2))
model.add(Conv2D(filters=64, kernel_size=4, padding='same', strides=1, activation='relu'))
model.add(MaxPool2D(pool_size=2))
model.add(Flatten())
model.add(Dense(512, activation='relu'))
model.add(Dense(10, activation='softmax'))
model.summary()
"""
_________________________________________________________________
Layer (type) Output Shape Param #
=================================================================
conv2d_1 (Conv2D) (None, 32, 32, 16) 784
_________________________________________________________________
max_pooling2d_1 (MaxPooling2 (None, 16, 16, 16) 0
_________________________________________________________________
conv2d_2 (Conv2D) (None, 16, 16, 32) 8224
_________________________________________________________________
max_pooling2d_2 (MaxPooling2 (None, 8, 8, 32) 0
_________________________________________________________________
conv2d_3 (Conv2D) (None, 8, 8, 64) 32832
_________________________________________________________________
max_pooling2d_3 (MaxPooling2 (None, 4, 4, 64) 0
_________________________________________________________________
flatten_1 (Flatten) (None, 1024) 0
_________________________________________________________________
dense_1 (Dense) (None, 512) 524800
_________________________________________________________________
dense_2 (Dense) (None, 10) 5130
=================================================================
Total params: 571,770
Trainable params: 571,770
Non-trainable params: 0
_________________________________________________________________
"""
간단한 모델을 구현하였습니다.
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
모델 컴파일을 진행합니다.
model.fit(X_train, Y_train, batch_size=150, epochs=1, validation_split=0.1)
"""
Train on 45000 samples, validate on 5000 samples
Epoch 1/1
45000/45000 [==============================] - 11s 248us/step - loss: 1.6029 - acc: 0.4202 - val_loss: 1.3092 - val_acc: 0.5284
"""
훈련을 진행합니다.
score = model.evaluate(X_test, Y_test)
print(score)
"""
10000/10000 [==============================] - 1s 78us/step
[1.3261255556106568, 0.5187]
"""
모델 평가를 진행합니다.
pred = model.predict(X_test)
label = ['airplane', 'automobile', 'bird', 'cat', 'deer', 'dog', 'frog', 'horse', 'ship', 'truck']
fig = plt.figure(figsize=(20,10))
for i, idx in enumerate(np.random.choice(X_test.shape[0], size=32)):
ax = fig.add_subplot(4,8, i+1 , xticks=[], yticks=[])
ax.imshow(X_test[i])
pred_idx = np.argmax(pred[idx])
true_idx = np.argmax(Y_test[idx])
ax.set_title("{}_{}".format(label[pred_idx], label[true_idx]), color='green' if pred_idx == true_idx else 'red')
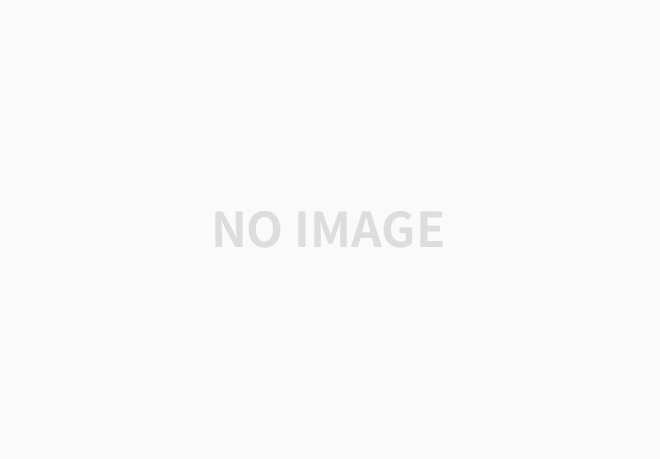
입력 데이터에 따라, 어떻게 예측을 했는 지에 대해 확인할 수 있습니다. 다양한 모델을 가지고 훈련을 진행해보고 결과를 위와 같이 확인해볼 수 있습니다. 이러한 방식으로 표현을 하면, 어떤한 데이터가 어떻게 예측을 했는 지, 유추해볼 수 있습니다.
반응형
'SW > Python' 카테고리의 다른 글
Python : Keras : Lstm : 오존 예측 : 예제, 사용법, 활용법 (0) | 2020.02.10 |
---|---|
Python : Keras : pretrain model : transfer learning : 활용, 예제, 방법 (0) | 2020.02.09 |
Python : Keras : 콘크리트 강도 분류 예측하기 : 예제, 방법, 컨셉 (0) | 2020.02.07 |
Python : Keras : iris 품종 예측하기 : 예제, 구현, 방법 (0) | 2020.02.06 |
Python : Keras : 사람의 정보로 사람의 수입 예측, 분류하기 : 예제 (0) | 2020.02.05 |